Identify customers to Mantle
When building a typical Shopify app and interacting with the Shopify API, you will need to generate a Shopify access_token for each merchant who installs your app using Shopify’s OAuth flow. The Shopify access token is required by Mantle so it can enrich your customer’s data and perform actions related to billing on the Shopify platform.
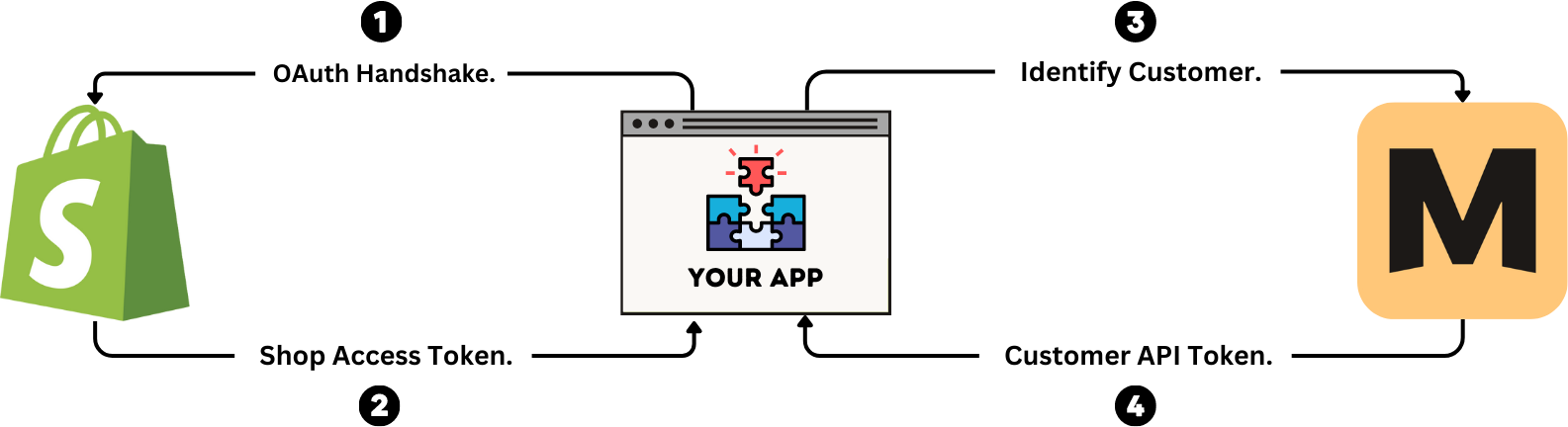
This part of the implementation is required to identify your customer with Mantle, which allows it to a form a base identity of the customer and provide Mantle with the customer’s Shopify access_token
.
When an app successfully identifies a customer with Mantle, the response will include a Mantle customer apiToken
. The role of the customer apiToken
is so your app can make secure requests to the Mantle servers, and the Mantle API can then perform whatever job was requested without, all while keeping your sensitive information safe.
It is recommended to store your Mantle Customer’s apiToken
in the database of your app’s shop/customer model alongside the Shopify access_token (if applicable), as this will be used by future requests to the Mantle API.
To identify your customers to Mantle, you will need to send a POST
request to the /identify
endpoint.
Although Mantle can generate a variety of accurate reports with just your Shopify Partner API credentials, it could still do more. By identifying your existing customers to Mantle, you can enrich your customer data to enable advanced segmentation and reporting.
How to identify your customers
There are two ways to identify your customers to Mantle:
- Identify customers using a cron job. You can identify your existing customers to Mantle using a cron job. This is the ideal solution if you’re not yet ready to implement Mantle Integrated and just want to enrich your data with things such as Shopify plan and customer location. Below is an example of how this could look if you’re using Node.js and Sequelize on your backend. We recommend you run this job on a schedule (e.g. once per day) to ensure your customer data is always up to date.
const MantleIdentifyTask = async () => {
// Fetch all active Shop records and loop through them
const shops = await Shop.findAll({
where: {
uninstalledAt: null,
},
});
for (let i = 0; i < shops.length; i++) {
const shop = shops[i];
try {
await identifyShop(shop);
} catch (error) {
console.log('[mantleIdentify] Something went wrong', error);
}
}
};
const identifyShop = async (shop) => {
const response = await fetch(`${process.env.MANTLE_API_URL}/identify`, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
Accept: 'application/json',
'X-Mantle-App-Id': process.env.MANTLE_APP_ID,
'X-Mantle-App-Api-Key': process.env.MANTLE_APP_API_KEY,
},
body: JSON.stringify({
platform: 'shopify',
platformId: shop.shopifyId,
myshopifyDomain: shop.myshopifyDomain,
accessToken: shop.accessToken,
}),
});
const result = await response.json();
await shop.update({ mantleApiToken: result.apiToken });
};
- Identify new or returning customers after the Shopify OAuth process. When a merchant installs your app (or reauthenticates with Shopify), you can make a
POST
request to the/v1/identify
endpoint to identify them to Mantle. This is the preferred way to do things if you’re using Mantle Integrated, as you’ll immediately be able to do things like surface plans and send usage events.
Example
Using mantle.js to identify the customer from the backend.
const mantleClient = new Mantle({
appId: process.env.MANTLE_APP_ID,
appApiKey: process.env.MANTLE_APP_API_KEY,
});
const shopData = await getShopDataFromShopify(session);
const mantleResponse = await mantleClient.identifyCustomer({
platform: "shopify",
accessToken: session.accessToken,
platformId: shopData.shop.id,
myshopifyDomain: session.shop,
email: shopData.shop.email,
name: shopData.shop.name,
});
await updateCustomerInYourDatabase(session, mantleResponse)
You can find a simple example of how to identify customers in the afterAuth function of our sample app which can be found on Github: mantle-shop-example.